Posted on 25 Jan 2013
I learned several Android tips the other day from an unlikely place: File->New Project
. I started a new project and found out that the latest SDK gives you an option to include an activity as your base. I chose LoginActivity and found several new things.
EditTexts can have errors
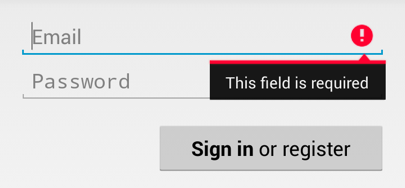
This is possible by calling setError
on the EditText.
LoginActivity.java - attemptLogin()
// Check for a valid password.
if (TextUtils.isEmpty(mPassword)) {
mPasswordView.setError(getString(R.string.error_field_required));
focusView = mPasswordView;
cancel = true;
} else if (mPassword.length() < 4) {
mPasswordView.setError(getString(R.string.error_invalid_password));
focusView = mPasswordView;
cancel = true;
}
The merge view
I’m still a little uncertain about this one, but after doing some searching it looks like you can speed up the display of your views if you use merge
. More info.
activity_login.xml
<merge xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".LoginActivity" >
<!-- Login progress -->
<LinearLayout ... />
<ScrollView ... />
</merge>
Value files can be layout specific
This one might be my favorite tip of them. I knew you could make resource files specific to versions of android, but I didn’t know you could make value files specific to a layout. With this you can stick layout specific strings in a separate file and not clutter up the main strings.xml
file.
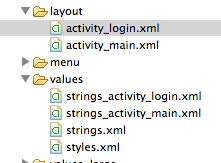
Posted on 17 Jan 2013
I have been integrating with outside services recently and decided to use webmock to stub the requests. Instead of creating a bunch of stub_request
for each section of the service you want to stub, you can just stub the entire domain to a rack app. It’s a lesser known feature of webmock because it’s just briefly mentioned in their readme.
You just have to call #to_rack
at the end of a stub_request
instead of #to_return
. In the rack app you can also validate that the requests you are sending contain the correct headers, are authenticated correctly, etc.
spec/spec_helper.rb
RSpec.configure do |config|
config.before do
stub_request(:any, /outsideservice\.com/).to_rack(OutsideServiceApp.new)
end
end
spec/support/outside_service_app.rb
class OutsideServiceApp
def call(env)
validate_request(env)
case [env["REQUEST_METHOD"], env["PATH_INFO"]]
when ["GET", "/orders"]
[200, {}, [File.read("spec/fixtures/orders.json")]]
else
[404, {}, []]
end
end
...
end
spec/outside_service_caller_spec.rb
describe OutsideServceCaller do
it "should load orders" do
OutsideServiceCaller.orders.should == File.read("spec/fixtures/orders.json")
end
end
Posted on 11 Jan 2013
I set up several EC2 instances earlier this week and had a lot of trouble trying to get them up and running. I figured I would share the things I learned, even if they seem pretty obvious.
When setting a specific kernel and ram id in AWS, the architecture should be chosen first.
I had to create an AMI from a specific snapshot and to do that you need to set the correct kernel and ram id. I could not find the one that I was supposed to use and eventually switched the architecture from 32 to 64, and suddenly the ids I was looking for were there.
If your instance is not responding, you might have picked the wrong kernel.
Since I was picking my own kernel id from a pretty huge select box, I managed to get it wrong. The instance launched and the AWS console said it couldn’t reach it via network. Loading it in the browser was broken as well. I tried launching new instances and rebooting several times to no avail. Eventually I checked the ids and they were off. Creating a new AMI with the correct kernel id fixed it.
If you need to transfer a file between instances, transfer between them directly.
This one might seem incredibly obvious, but for some reason I didn’t think to do this until after I was half way through downloading a 3GB+ file. Once I realized it, I started the transfer between them directly and the transfer between them finished before my download did.
Posted on 19 Dec 2012
I have been writing an ncurses campfire client hobostove and recently gave a presentation on using Ncurses and Ruby.
Hobostove uses the ncurses-ruby gem. It’s pretty old but so is Ncurses. Here I’ll give the basics of setting up, refreshing, and tearing down. I’ll do a panel usage post later.
Initialization
Ncurses.initscr
Ncurses.cbreak
Ncurses.noecho
Ncurses.initscr
- sets up Ncurses
Ncurses.cbreak
- don’t wait for a new line to send characters to Ncurses.getch
Ncurses.noecho
- don’t echo typed characters to the screen
Update
Ncurses.doupdate
Ncurses.refresh
Ncurses.doupdate
- compares the virtual screen to the physical screen and updates accordingly
Ncurses.refresh
- writes to the terminal
Tear down
Ncurses.echo
Ncurses.endwin
Ncurses.echo
- echos characters back to the screen
Ncurses.endwin
- stops ncurses
Resources
- TLDP Ncurses How To
Posted on 12 Dec 2012
I have been creating a gem recently and will usually want to open up an irb
session to play around with ideas. When opening the irb
session I had to configure the gem and it started to get annoying. I got envious of rails console
and decided to make my own script/console
for the gem.
Below is what it ended up looking like.
script/console
b = File.expand_path('../../lib/', __FILE__)
$:.unshift lib unless $:.include?(lib)
require 'my_gem'
require 'irb'
MyGem.configure do |config|
config.name = "My Gem"
end
IRB.setup(nil)
irb = IRB::Irb.new
IRB.conf[:MAIN_CONTEXT] = irb.context
irb.context.evaluate("require 'irb/completion'", 0)
trap("SIGINT") do
irb.signal_handle
end
catch(:IRB_EXIT) do
irb.eval_input
end